9 React and JavaScript Tips You Should Start Using Today
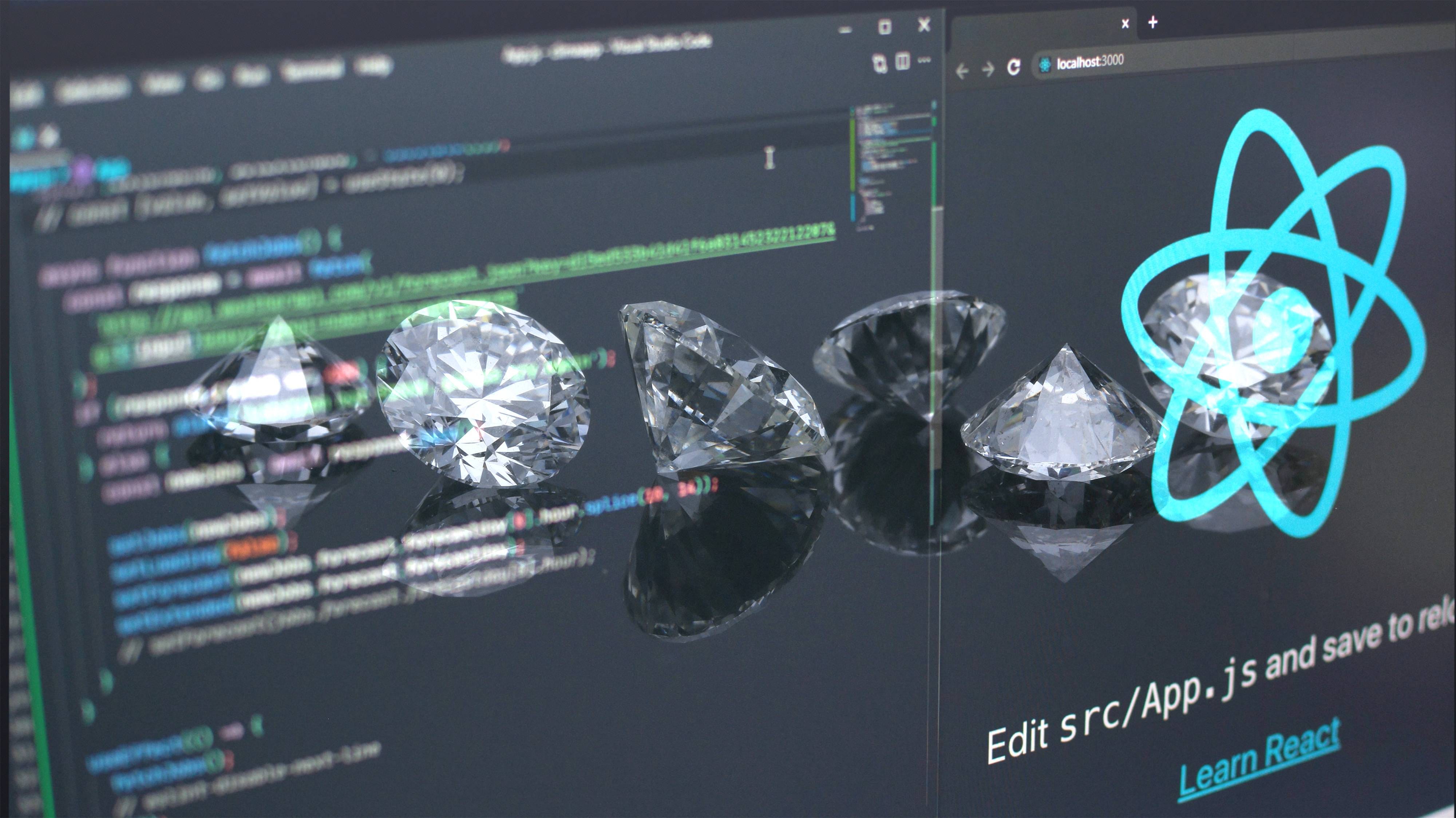
Although this guide is intended for React and JavaScript beginners, it isn’t limited to them. In fact, I’m certain many of the following nine React and JavaScript tips will be helpful to beginners and experts alike.
If you're a seasoned coder, implement these hacks to become more productive and improve your code. If you're new to coding, this is your chance to master React and JavaScript from the very beginning.
React Tips
1. setState() using function composition
By using function composition, you can compose functions for different purposes. This approach also helps you to write cleaner and more readable code.
Have you worked with forms before? I bet you have.
When it comes to forms, you may need to handle several input fields. A common solution is to create a setYourFieldName
setter function for each input field so you can update its value.
Something like the following:
const [firstName, setFirstName] = useState('');
const [lastName, setLastName] = useState('');
const [username, setUsername] = useState('');
setFirstName("blarz");
...
While the above is valid, it isn't elegant. For a cleaner way of doing it, try using function composition.
Let’s take a quick look at this scrawl on my whiteboard. Try to read it line by line.
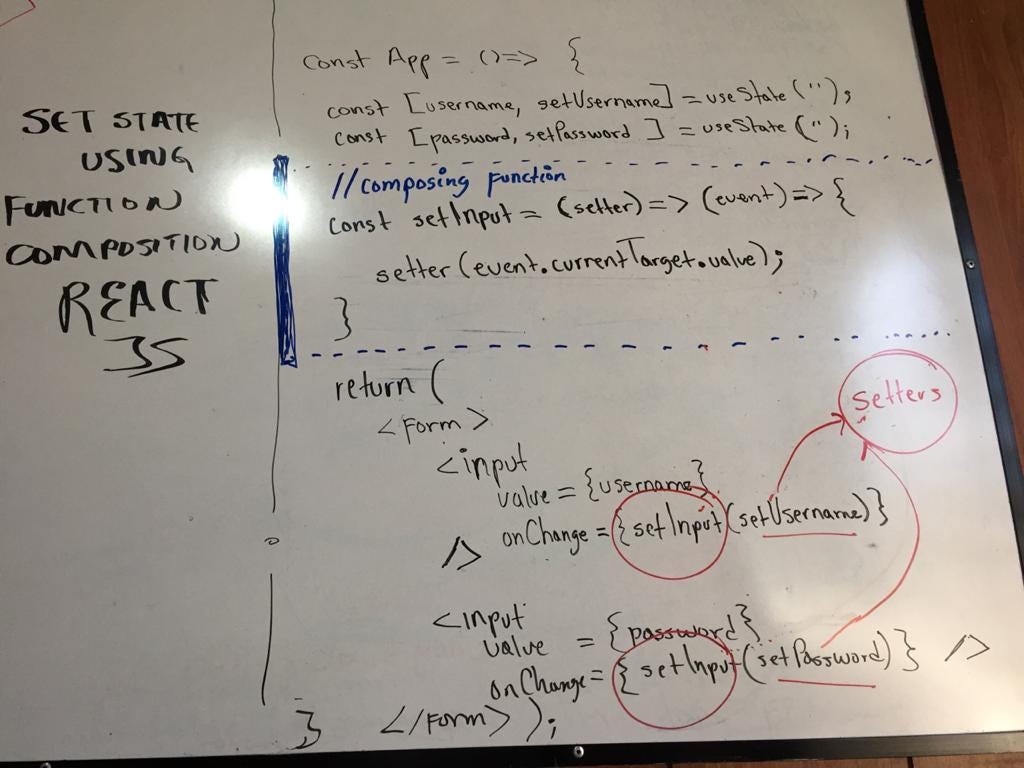
I am sorry if you weren't able to read it. (If you can't read my handwriting, cut me some slack, I use a keyboard for a living, not a marker 😜). The idea with this is to delay the reading of each line in order to give your brain time to digest it effortlessly.
For help mastering function composition, take a look at the live version of my example here.
To sum this up, using function composition allows you to:
- Write cleaner and more readable code.
- Centralize the logic for updating the state.
- Work with any number of input fields.
2. Pass props down using the spread operator
Components communicate with other components via props. Props are data (or settings) that components need. This means you may need to pass down a list of them.
It is just fine to pass a few props down explicitly and individually, but when you need to pass a huge list of them, it can be a pain.
Here is when the spread operator (...) comes to the rescue.
The simplest way to pass several props at once is to define an object with all the props the component needs. So you don’t need to be explicit setting the prop name and its corresponding value.
Let’s see the next piece of code for a better understanding.
As you can see in the code snippet, the Logo component expects four props: isBrandText
, brandImageUrl
, altAttr
, and brandText
. So in the app component, we pass down thelogoInfo
object, which is spreading its properties and the corresponding values—a powerful and clean solution!
3. Using short-circuit evaluation
The short-circuit evaluation makes our code more elegant, readable, and clean. This tip sounds simple, but continue reading to see a few pitfalls you might not have seen before.
Let’s take a look at the next example.
return isLoading && <SpinnerComponent/>;
The above code checks if isLoading is true. If so, it renders the SpinnerComponent ; otherwise, it does nothing.
What will be the output of the following return statement in the ConditionalsInReactcomponent ?
Did you guess it? The output will be the following:
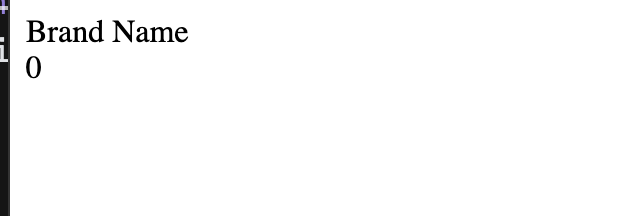
What happened here? Why is it rendering 0? Let’s discuss it.
🚨 Values of renderables and non-renderables in React
I spent my precious time trying to understand why the evaluation was rendering 0.
The answer:
I discovered that React will render anything that is a type of string or number, but it will not render value types of false
, null
, or undefined
.
For a better understanding, let’s take a look at the next statements and their outputs. You will notice how the above explanation now makes sense.
return 1 && <Spinner/> // it will render <Spinner/>
return "string" && <Spinner/> // it will render <Spinner/>
return 0 && <Spinner/> // it will render 0
Solution
To make a short-circuit evaluation safe, you can use one of two strategies: the ternary operator or double negation (double casting).
The ternary operator
{rating ? : null }
Double negation
You can do something like {!!rating && }
, making sure the rating is cast to a Boolean value.
4. Update multiple-input state values with only ONE handler
When you're working with forms in React, you will need to watch for changes in the input fields. When there are only a few input fields, you can easily catch those changes by creating an updater function for each of them.
However, if you're working with large forms with several input fields, this approach would be a bad idea.
Instead, define one handler function to use for all input fields. By doing this, you reduce the lines of code, which in turn makes you more productive.
Let’s take a look at the next component.
In the above code snippet, we have created a component called MultipleInputs, which basically renders a form with several input fields.
Then we have declared the state variable inputs and its corresponding updater function setInputs. The inputs variable is the object with all the input fields we are going to need— firstName
, lastName
, username
, email
, password
, etc.
Now let’s focus on the handleChange function. Here we are receiving an input e
, which is a SyntheticEvent object. This object contains useful metadata such as the target input’s ID, name, and current value.
Let’s deconstruct the e
object and extract the name and value from its properties.
const handleChange = (e) => {
...
const { name, value } = e.target;
...
};
Finally, we update the current input field by passing its name as a dynamic key [name]
and its corresponding value.
const handleChange = (e) => {
const { name, value } = e.target;
setInputs((prevState) => ({ ...prevState, [name]: value })); };
Voila! You have saved time and have cleaner code.
5. Modify arrays in React with one of three methods
Below, I've demonstrated the three best approaches to modify arrays in React. Take a look at the scrawls on my whiteboard and read them line by line.
Using Lifecycle or setState()
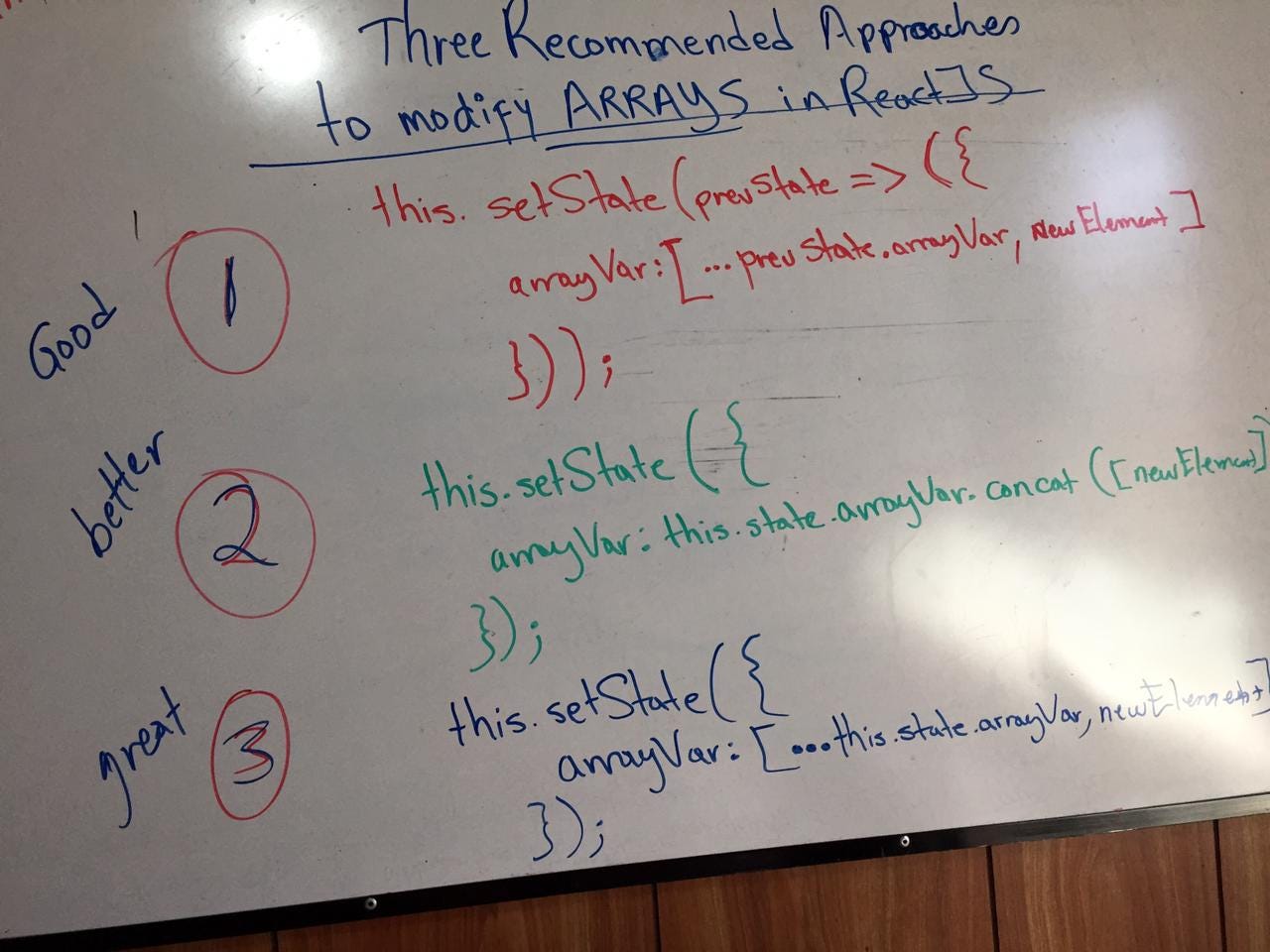
Using Hooks — short version
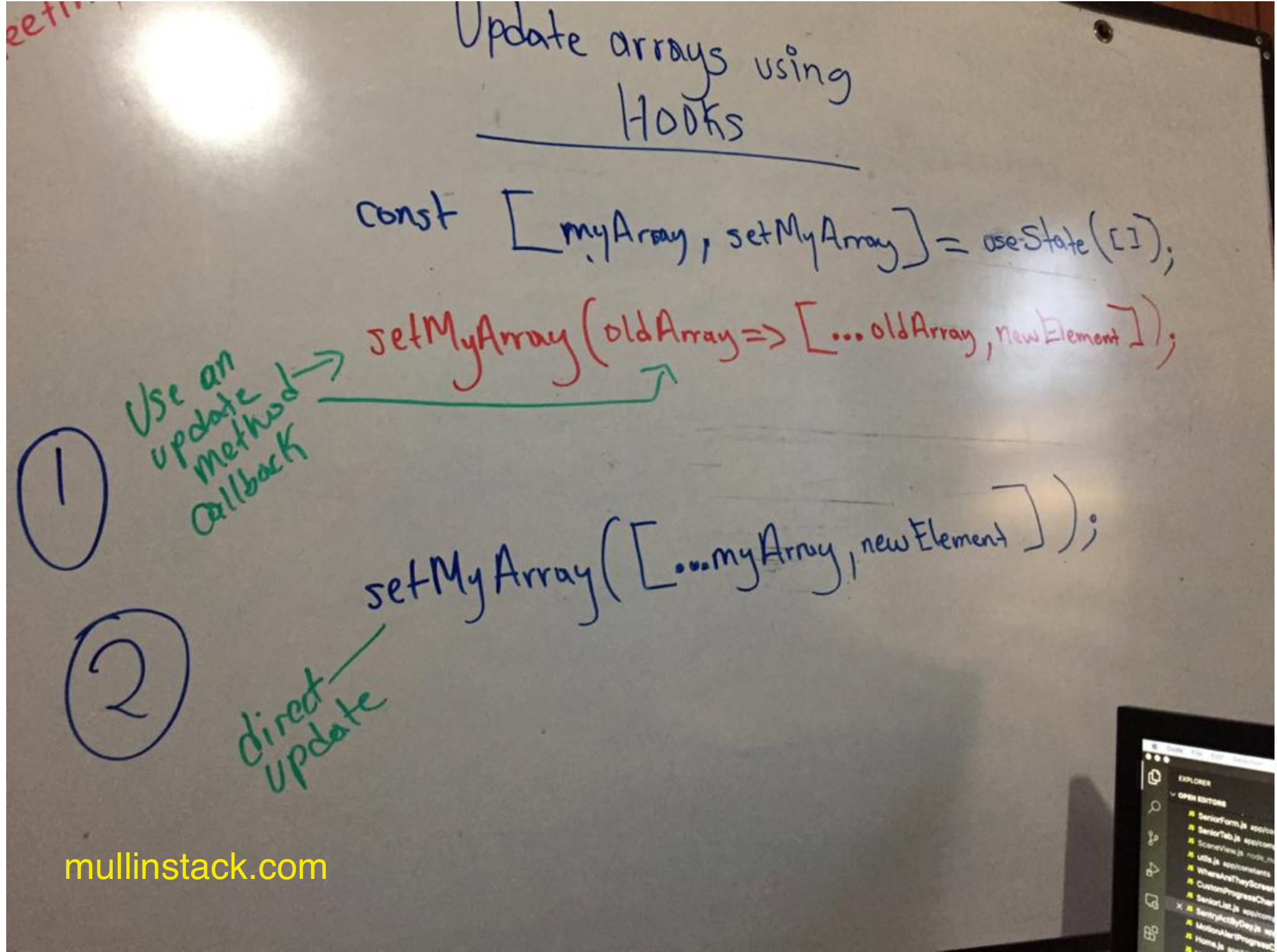
Using Hooks — detailed version
Next I will show you a real example using Hooks. If you want to see how this should be done using class-based components, scroll up a bit to the picture of my scrawl whiteboard.
JavaScript Tips
6. Pause Console Debugger
One day, I was fighting with a dropdown. I just wanted to inspect one of its elements. It was frustrating.
The dropdown closed automatically when I tried to right-click on the element and click on the “Inspect” option.
I bet you have experienced this headache before too, but from now on, you will have the antidote:
- Open your console from a browser.
- Type in the following command:
setTimeout(() => {debugger;}, 5000)
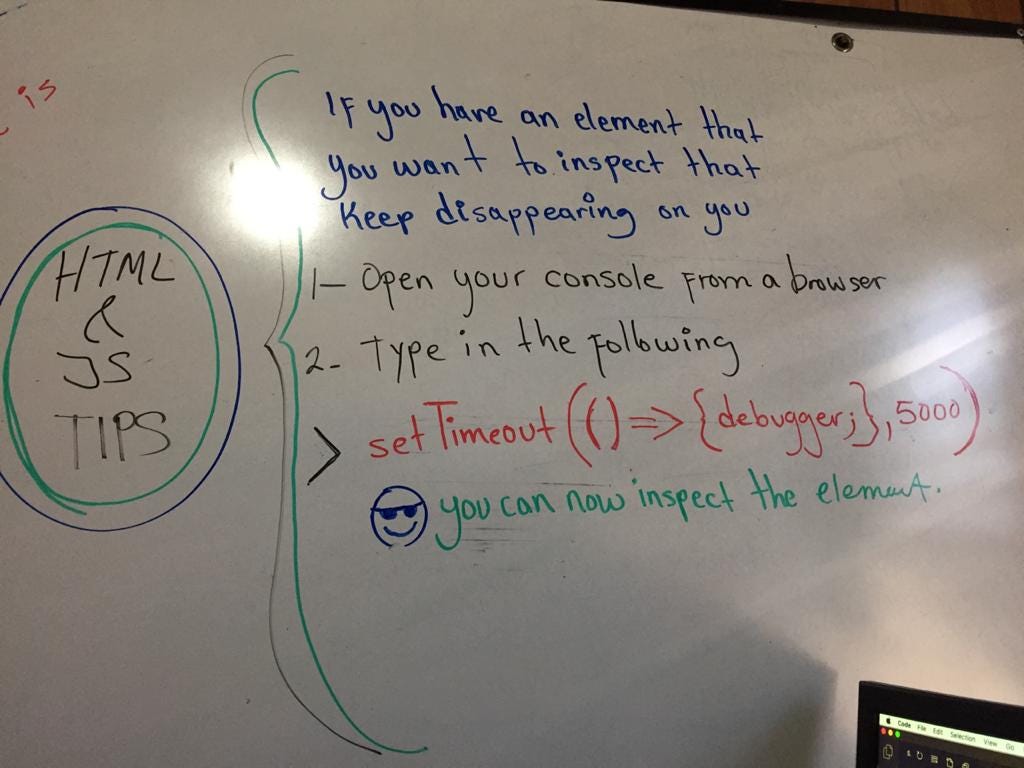
I hope this comes in handy!
7. Welcome back to console.log()
For those who are like me and always come back to the console.log()
, here's a good tip: Type in the code below, and you will see the variable name and its value.
console.log({yourVariable});
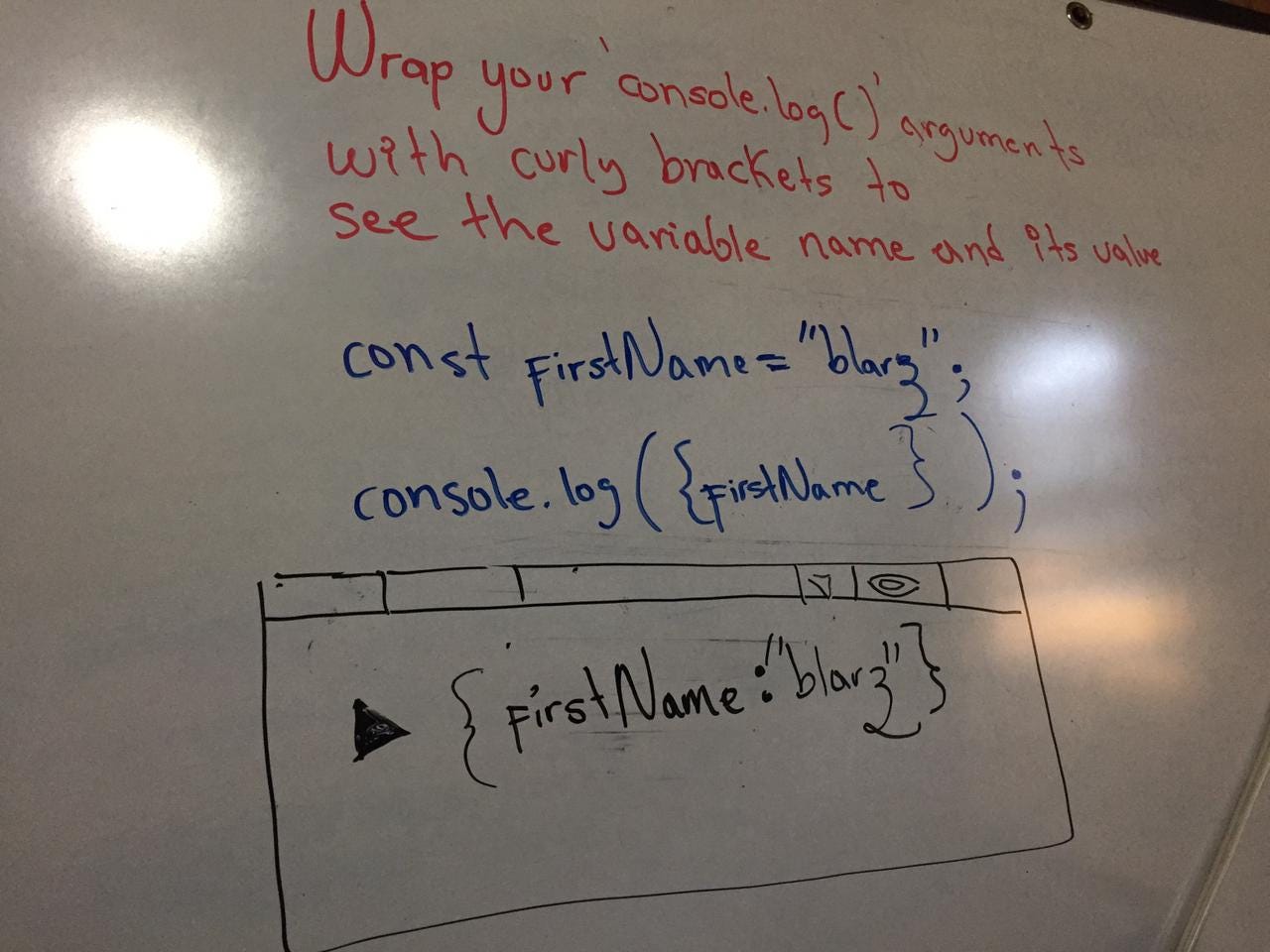
8. Let React assign keys to children
Assigning keys to children is an important matter in React. It’s the way React identifies which items have changed.
I have used several methods to assign unique keys to children of an element, but once I discovered that React.Children.toArray
has the power of assigning keys to children, my life changed.
Here's an explanation of React.Children.toArray
from the official React doc:
Returns the children opaque data structure as a flat array with keys assigned to each child. Useful if you want to manipulate collections of children in your render methods, especially if you want to reorder or slice this.props.children before passing it down.
It’s really simple. You just need to wrap the children, something like the code below.
9. Use the nullish coalescing operator
Using a fallback value with the OR (||) operator is a good idea when it comes to check if a value is falsy.
Quick refresher — JavaScript falsy values:
false, '', 0, "", undefined, null, NaN
Let’s suppose we have this Car component.
Did you notice the issue in the above code?
In the span tag, we are using a fallback value whenever the car.price
statement is evaluated as falsy. So this means if the car.price
is 0, the component will render “Not available.” This, of course, is wrong because we should display 0 as the price.
The above code doesn’t cover the special case in which we need to check if the value is either null
or undefined
.
Solution
Here is when the nullish coalescing operator ??
comes in handy.
This is a logical operator that returns its right-hand side operand when its left-hand side operand is null or undefined .
Now, using the nullish operator, we will have the following:
Full code
You can find all React files/components on this Codesandbox.
Final Thoughts
I am sure that more than one of the nine React and JavaScript tips we discussed throughout this post will save you time and make you more productive from now on.
Start off using function composition when you want to compose a function for different purposes.
And remember, one of the main patterns in React is the usage of props to communicate to components, so when it comes to passing a huge list of props down, use the spread operator (...) to pass it all at once.
Finally, if you follow these tips you will be able to write cleaner, more readable, and elegant code.
I hope this post turned be out helpful for you. If you enjoyed it, please share it to reach a wider audience.
Thanks for reading. See you in the next piece.